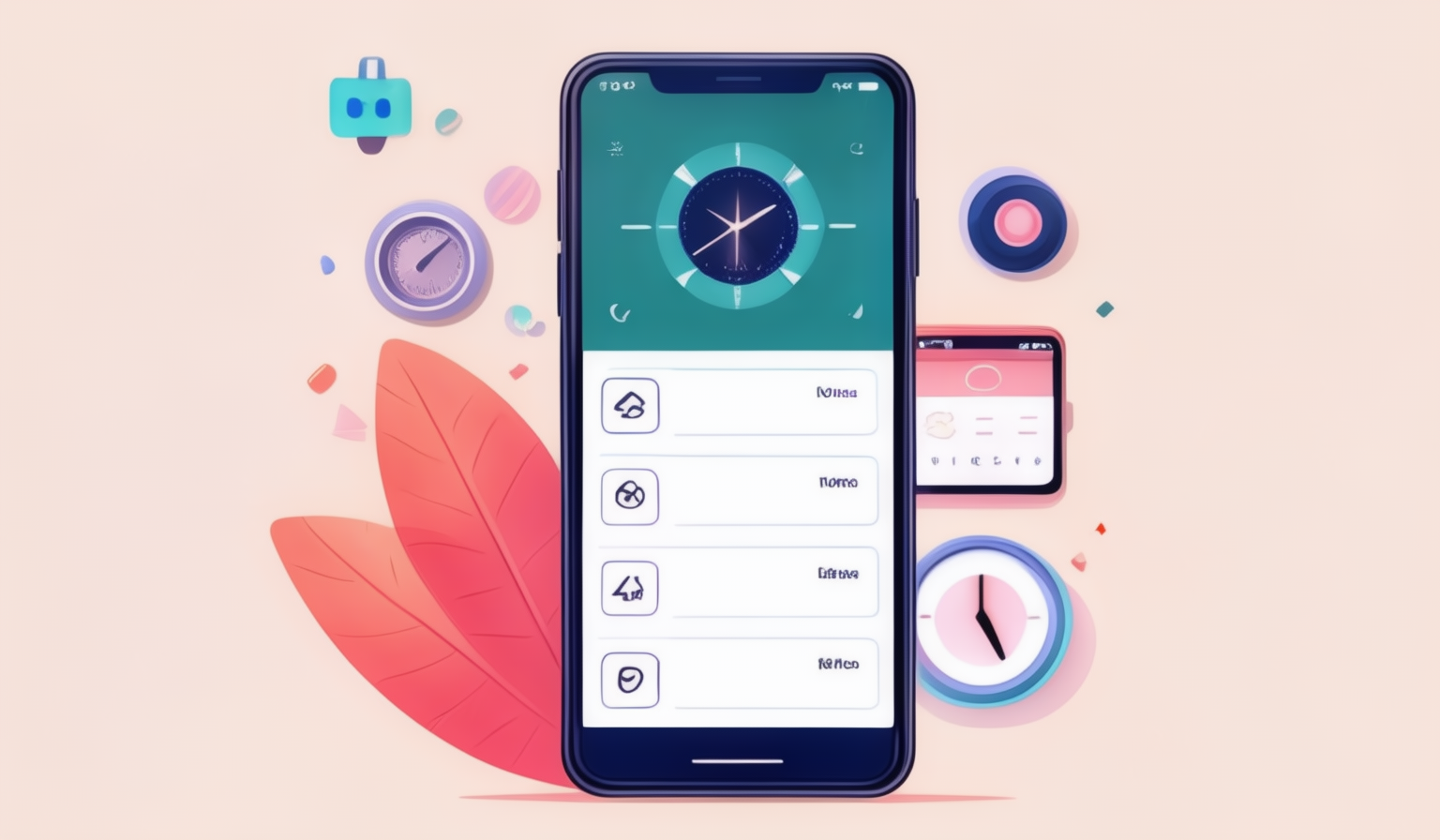
Seeking ways to schedule the execution of Flutter Android code in the background post-app termination? Let’s explore how you can achieve this in your Flutter app development journey!
Table of Contents:
- Applications of Background Execution
- Various Approaches
- Implementation
- Example Use Case
- Disabling Battery Optimization
- Conclusion
Applications of Background Execution
Periodic Maintenance:
For Flutter app development Background scheduling is helpful for routine maintenance operations like app content updates, data cleanups from caches, and background analytics. This keeps the application current and functional.
Notifications and Messaging:
Push notifications, background messaging, and other real-time changes require background jobs to be managed. Your app can react to events or incoming messages even while it’s not active by scheduling tasks to run in the background.
Data Synchronization:
Background activities are frequently employed to synchronize data. This includes making sure the program has the most recent data even when it is not in use, updating local data stores, and retrieving and processing data from remote servers.
Security and Authentication:
Security-related tasks, like renewing authentication tokens, scanning for security updates, or guaranteeing the integrity of user credentials, can frequently be handled by background tasks.
Various Approaches
There are numerous ways/packages we can use to schedule alarms for executing code in the background. Let’s explore a few, and then we’ll dive into one of the approaches in detail.
flutter_background:
flutter_background plugin keeps the Flutter app isolated and running in the background. It’s the simplest way to keep the Flutter app up and running even after the app is terminated. It shows customizable notifications as well to notify users and that can be useful in apps like Chat-App where users are notified when receiving messages in the background.
flutter_background_service:
flutter_background_service plugin uses a separate isolate for executing code in the background. It also shows customizable notifications. Since the service uses isolates, you won’t be able to share references between UI and Service, you can perform such operations using methods provided by the plugin.
android_alarm_manager_plus:
android_alarm_manager_plus plugin allows not only code execution in the background but also different schedules and periodic triggers. Using this plugin, one can set the exact time when code should be executed regardless of whether the app is in the foreground or background.
It also uses an exact alarm to trigger a call back at a precise time in the future. This plugin offers many functionalities to do the task, and due to this reason, we’ll deep dive into it in the next phase.
Implementation
Now that we have seen different approaches to background scheduling, we’ll be implementing one of the above packages from the pub. dev which is android_alarm_manager_plus
1. Add Dependency:
In your Flutter project’s pubspec.yaml file, add the android_alarm_manager_plus package as a dependency:
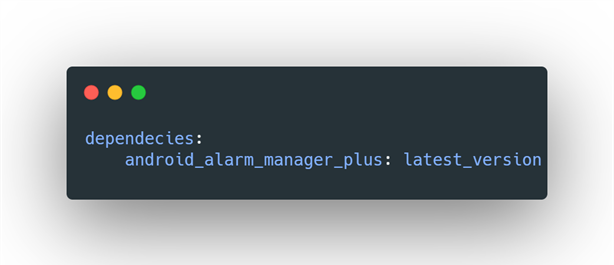
Replace “latest_version” with the latest version of the android_alarm_manager_plus package.
2. Add required permissions:
After importing this plugin to your project as usual, add the following to your AndroidManifest.xml within the <manifest></manifest> tags:
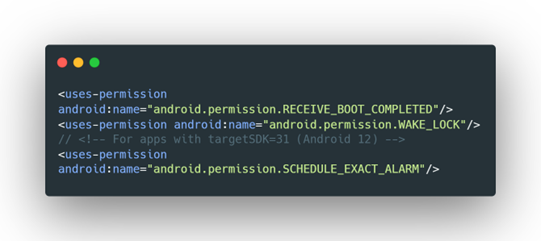
3. Add Services and Broadcast Receivers:
Next, within the <application></application> tags, add:

4. Initialization:
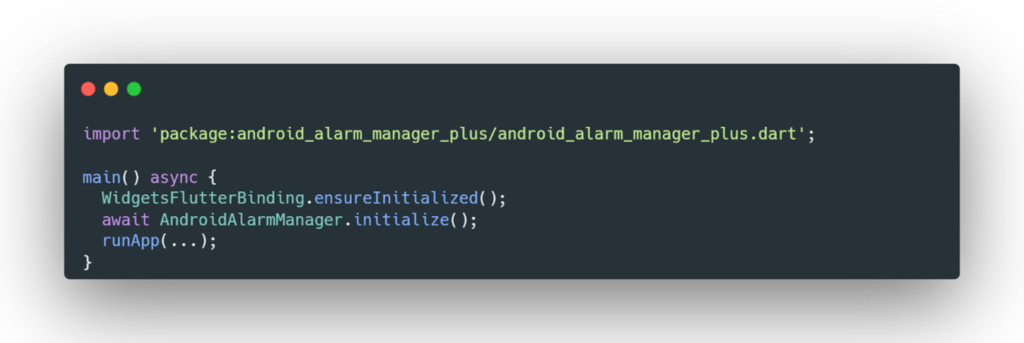
5. Usage:
There are three ways or methods in which we can implement scheduling regardless of whether the application is in the background or foreground:
i. AndroidAlarmManager.oneShot()
- Schedules a one-shot timer to run ‘callback’ after time ‘delay’.
- It will run in Isolate owned by the AndroidAlarmManager service.
- ‘callback must be either a top-level function or a static method from the class
- If `exact` is passed as ‘true’ (default is false), the timer will be created with Android’s ‘AlarmManagerCompat.setExact’. For apps with ‘targetSDK=31’ before scheduling an exact alarm, a check for ‘SCHEDULE_EXACT_ALARM’ permission is required which we’ve added in ‘step 2’ of Implementation. Otherwise, an exception will be thrown, and the alarm won’t be scheduled.

ii. AndroidAlarmManager.oneShotAt()
- Schedules a one-shot timer to run ‘callback’ at ‘time’.
- All the parameters in this function work the same as explained above, just the difference being is that ‘callback’ is executed at a given ‘time’.
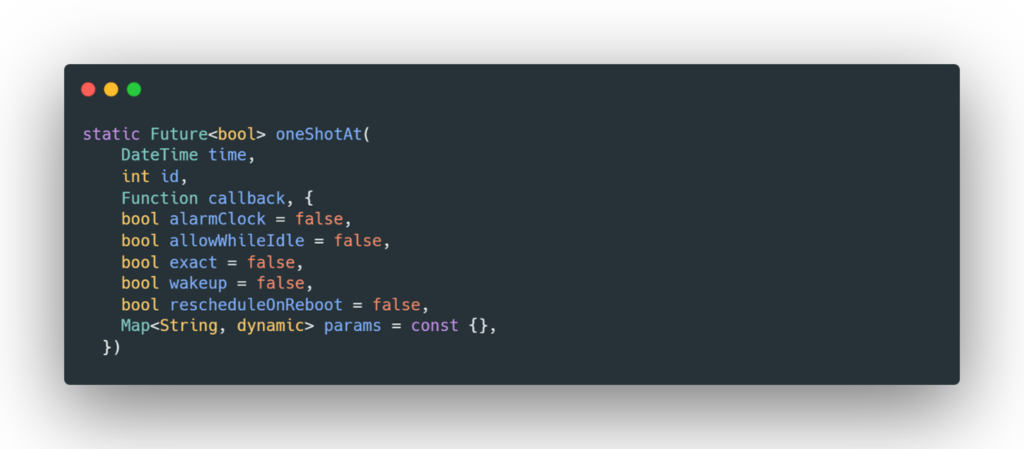
iii. AndroidAlarmManager.periodic()
- Schedules a repeating timer to run ‘callback’ with period ‘duration’.
- If ‘start’ is passed, the timer will first go off at that time and subsequently run with period ‘duration’.
- All the parameters in this function work the same as explained above
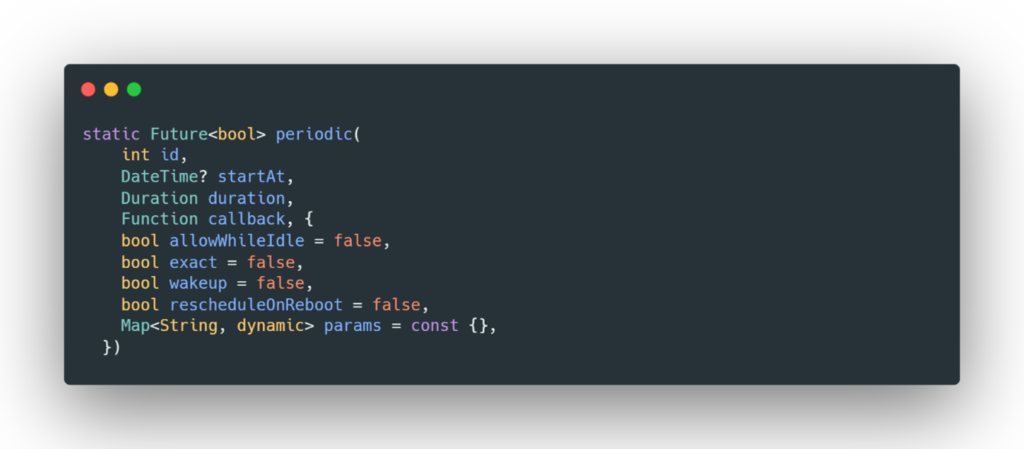
Example Use Case
Let’s take an example usage of the periodic method in Flutter app development. You’re developing a weather forecast app for a client who depends on app to schedule his/her outdoor activities. The app utilizes background code scheduling to update weather data twice a day. Even if the app is closed, user receives timely notifications about weather changes.
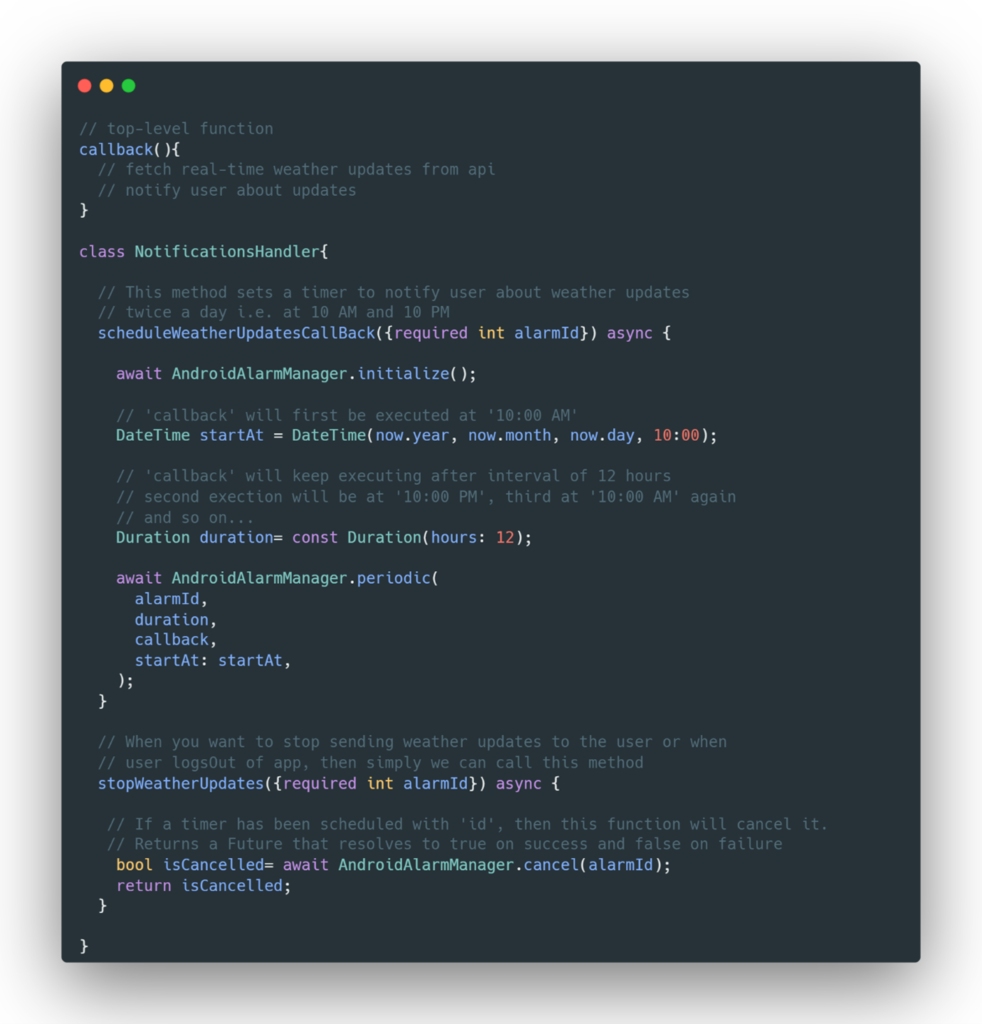
Disabling Battery Optimization
Disabling battery optimization in flutter app development is a crucial part of scheduling as it can disrupt scheduled alarms which can lead to problems like delayed execution of schedule or simply alarms not being executed at all.
Check out dont-kill-my-app to find out more about optimizations performed by different vendors and how to disable them manually.
To disable battery optimization programmatically, use the below package from pub.dev:
- disable_battery_optimization: This package lets you prompt a dialogue in your app, when confirmed, it disables battery optimization for your app without navigating to any other screen.
Conclusion
To sum up, Flutter’s implementation of background code execution is essential for improving the usability and functionality of the app. Through a variety of techniques, including isolates, and plugins, one can carry out operations without interruption even when the application is running in the background.
To ensure minimal impact on battery life and device performance, it is crucial to take battery optimization strategies into account. You may provide a smoother and more responsive user experience by carefully managing background operations and following best practices. This approach aids in achieving a balance between functionality and resource efficiency in your flutter app development endeavors.